test(ivy): run view_injector_integration tests on node (#28593)
There is nothing browser specific in those tests and fakeAsync is supported on node. Testing / debugging on node is often faster than on Karma. PR Close #28593
This commit is contained in:
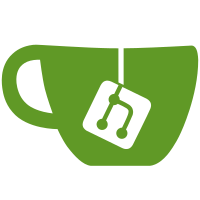
committed by
Miško Hevery
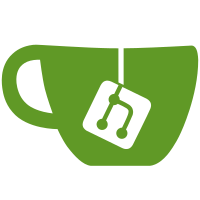
parent
6e4ef91ceb
commit
0e4705aec3
@ -8,7 +8,6 @@
|
||||
|
||||
import {Attribute, ChangeDetectionStrategy, ChangeDetectorRef, Component, ComponentFactoryResolver, DebugElement, Directive, ElementRef, EmbeddedViewRef, Host, Inject, InjectionToken, Injector, Input, NgModule, Optional, Pipe, PipeTransform, Provider, Self, SkipSelf, TemplateRef, Type, ViewContainerRef} from '@angular/core';
|
||||
import {ComponentFixture, TestBed, fakeAsync} from '@angular/core/testing';
|
||||
import {getDOM} from '@angular/platform-browser/src/dom/dom_adapter';
|
||||
import {expect} from '@angular/platform-browser/testing/src/matchers';
|
||||
import {ivyEnabled, modifiedInIvy, obsoleteInIvy, onlyInIvy} from '@angular/private/testing';
|
||||
|
||||
@ -183,8 +182,7 @@ export class DuplicatePipe2 implements PipeTransform {
|
||||
class TestComp {
|
||||
}
|
||||
|
||||
(function() {
|
||||
function createComponentFixture<T>(
|
||||
function createComponentFixture<T>(
|
||||
template: string, providers?: Provider[] | null, comp?: Type<T>): ComponentFixture<T> {
|
||||
if (!comp) {
|
||||
comp = <any>TestComp;
|
||||
@ -194,19 +192,15 @@ class TestComp {
|
||||
TestBed.overrideComponent(comp !, {add: {providers: providers}});
|
||||
}
|
||||
return TestBed.createComponent(comp !);
|
||||
}
|
||||
}
|
||||
|
||||
function createComponent(
|
||||
template: string, providers?: Provider[], comp?: Type<any>): DebugElement {
|
||||
function createComponent(template: string, providers?: Provider[], comp?: Type<any>): DebugElement {
|
||||
const fixture = createComponentFixture(template, providers, comp);
|
||||
fixture.detectChanges();
|
||||
return fixture.debugElement;
|
||||
}
|
||||
|
||||
describe('View injector', () => {
|
||||
// On CJS fakeAsync is not supported...
|
||||
if (!getDOM().supportsDOMEvents()) return;
|
||||
}
|
||||
|
||||
describe('View injector', () => {
|
||||
const TOKEN = new InjectionToken<string>('token');
|
||||
|
||||
beforeEach(() => {
|
||||
@ -260,8 +254,7 @@ class TestComp {
|
||||
it('should instantiate providers that have dependencies with SkipSelf', () => {
|
||||
TestBed.configureTestingModule({declarations: [SimpleDirective, SomeOtherDirective]});
|
||||
TestBed.overrideDirective(
|
||||
SimpleDirective,
|
||||
{add: {providers: [{provide: 'injectable1', useValue: 'injectable1'}]}});
|
||||
SimpleDirective, {add: {providers: [{provide: 'injectable1', useValue: 'injectable1'}]}});
|
||||
TestBed.overrideDirective(SomeOtherDirective, {
|
||||
add: {
|
||||
providers: [
|
||||
@ -322,9 +315,7 @@ class TestComp {
|
||||
];
|
||||
TestBed.overrideDirective(SimpleDirective, {set: {providers}});
|
||||
const el = createComponent('<div simpleDirective></div>');
|
||||
expect(el.children[0].injector.get('injectable1')).toEqual([
|
||||
'injectable11', 'injectable12'
|
||||
]);
|
||||
expect(el.children[0].injector.get('injectable1')).toEqual(['injectable11', 'injectable12']);
|
||||
});
|
||||
|
||||
it('should instantiate providers lazily', () => {
|
||||
@ -516,8 +507,7 @@ class TestComp {
|
||||
() => {
|
||||
TestBed.configureTestingModule({declarations: [SimpleComponent, NeedsService]});
|
||||
TestBed.overrideComponent(
|
||||
SimpleComponent,
|
||||
{set: {viewProviders: [{provide: 'service', useValue: 'service'}]}});
|
||||
SimpleComponent, {set: {viewProviders: [{provide: 'service', useValue: 'service'}]}});
|
||||
expect(() => createComponent('<div simpleComponent needsService></div>'))
|
||||
.toThrowError(/No provider for service!/);
|
||||
});
|
||||
@ -526,8 +516,7 @@ class TestComp {
|
||||
() => {
|
||||
TestBed.configureTestingModule({declarations: [SimpleComponent, NeedsService]});
|
||||
TestBed.overrideComponent(
|
||||
SimpleComponent,
|
||||
{set: {viewProviders: [{provide: 'service', useValue: 'service'}]}});
|
||||
SimpleComponent, {set: {viewProviders: [{provide: 'service', useValue: 'service'}]}});
|
||||
expect(() => createComponent('<div simpleComponent><div needsService></div></div>'))
|
||||
.toThrowError(/No provider for service!/);
|
||||
});
|
||||
@ -558,8 +547,7 @@ class TestComp {
|
||||
SimpleComponent, {set: {providers: [{provide: 'service', useValue: 'hostService'}]}});
|
||||
TestBed.overrideComponent(SimpleComponent, {set: {template: '<div needsService></div>'}});
|
||||
const el = createComponent('<div simpleComponent></div>');
|
||||
expect(el.children[0].children[0].injector.get(NeedsService).service)
|
||||
.toEqual('hostService');
|
||||
expect(el.children[0].children[0].injector.get(NeedsService).service).toEqual('hostService');
|
||||
});
|
||||
|
||||
it('should instantiate directives that depend on view providers of a component', () => {
|
||||
@ -568,16 +556,14 @@ class TestComp {
|
||||
SimpleComponent, {set: {providers: [{provide: 'service', useValue: 'hostService'}]}});
|
||||
TestBed.overrideComponent(SimpleComponent, {set: {template: '<div needsService></div>'}});
|
||||
const el = createComponent('<div simpleComponent></div>');
|
||||
expect(el.children[0].children[0].injector.get(NeedsService).service)
|
||||
.toEqual('hostService');
|
||||
expect(el.children[0].children[0].injector.get(NeedsService).service).toEqual('hostService');
|
||||
});
|
||||
|
||||
it('should instantiate directives in a root embedded view that depend on view providers of a component',
|
||||
() => {
|
||||
TestBed.configureTestingModule({declarations: [SimpleComponent, NeedsService]});
|
||||
TestBed.overrideComponent(
|
||||
SimpleComponent,
|
||||
{set: {providers: [{provide: 'service', useValue: 'hostService'}]}});
|
||||
SimpleComponent, {set: {providers: [{provide: 'service', useValue: 'hostService'}]}});
|
||||
TestBed.overrideComponent(
|
||||
SimpleComponent, {set: {template: '<div *ngIf="true" needsService></div>'}});
|
||||
const el = createComponent('<div simpleComponent></div>');
|
||||
@ -771,8 +757,7 @@ class TestComp {
|
||||
})
|
||||
.createComponent(MyComp);
|
||||
|
||||
expect(compFixture.componentInstance.vc.parentInjector.get('someToken'))
|
||||
.toBe('someValue');
|
||||
expect(compFixture.componentInstance.vc.parentInjector.get('someToken')).toBe('someValue');
|
||||
});
|
||||
});
|
||||
|
||||
@ -906,8 +891,7 @@ class TestComp {
|
||||
it('should inject ViewContainerRef', () => {
|
||||
TestBed.configureTestingModule({declarations: [NeedsViewContainerRef]});
|
||||
const el = createComponent('<div needsViewContainerRef></div>');
|
||||
expect(
|
||||
el.children[0].injector.get(NeedsViewContainerRef).viewContainer.element.nativeElement)
|
||||
expect(el.children[0].injector.get(NeedsViewContainerRef).viewContainer.element.nativeElement)
|
||||
.toBe(el.children[0].nativeElement);
|
||||
});
|
||||
|
||||
@ -982,8 +966,7 @@ class TestComp {
|
||||
});
|
||||
const el = createComponent(
|
||||
'<div [simpleDirective]="true | pipeNeedsChangeDetectorRef" directiveNeedsChangeDetectorRef></div>');
|
||||
const cdRef =
|
||||
el.children[0].injector.get(DirectiveNeedsChangeDetectorRef).changeDetectorRef;
|
||||
const cdRef = el.children[0].injector.get(DirectiveNeedsChangeDetectorRef).changeDetectorRef;
|
||||
expect(el.children[0].injector.get(SimpleDirective).value.changeDetectorRef).toEqual(cdRef);
|
||||
});
|
||||
|
||||
@ -1027,11 +1010,8 @@ class TestComp {
|
||||
class SomeComponent {
|
||||
}
|
||||
|
||||
@NgModule({
|
||||
declarations: [SomeComponent],
|
||||
exports: [SomeComponent],
|
||||
entryComponents: [SomeComponent]
|
||||
})
|
||||
@NgModule(
|
||||
{declarations: [SomeComponent], exports: [SomeComponent], entryComponents: [SomeComponent]})
|
||||
class SomeModule {
|
||||
}
|
||||
|
||||
@ -1065,16 +1045,14 @@ class TestComp {
|
||||
imports: [SomeModule],
|
||||
declarations: [ComponentThatLoadsAnotherComponentThenMovesIt],
|
||||
});
|
||||
const fixture =
|
||||
createComponentFixture(`<listener-and-on-destroy></listener-and-on-destroy>`);
|
||||
const fixture = createComponentFixture(`<listener-and-on-destroy></listener-and-on-destroy>`);
|
||||
fixture.detectChanges();
|
||||
|
||||
// This test will fail if the ngOnInit of ComponentThatLoadsAnotherComponentThenMovesIt
|
||||
// throws an error.
|
||||
});
|
||||
});
|
||||
});
|
||||
})();
|
||||
});
|
||||
|
||||
class TestValue {
|
||||
constructor(public value: string) {}
|
||||
|
Reference in New Issue
Block a user