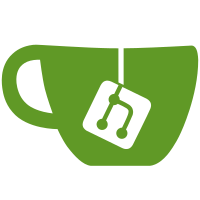
Subclassing errors is problematic since Error returns a new instance. All of the patching which we do than prevent proper application of source maps. PR Close #14160
49 lines
1.4 KiB
TypeScript
49 lines
1.4 KiB
TypeScript
/**
|
|
* @license
|
|
* Copyright Google Inc. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
import {Pipe, PipeTransform} from '@angular/core';
|
|
import {invalidPipeArgumentError} from './invalid_pipe_argument_error';
|
|
|
|
/**
|
|
* @ngModule CommonModule
|
|
* @whatItDoes Generic selector that displays the string that matches the current value.
|
|
* @howToUse `expression | i18nSelect:mapping`
|
|
* @description
|
|
*
|
|
* Where `mapping` is an object that indicates the text that should be displayed
|
|
* for different values of the provided `expression`.
|
|
* If none of the keys of the mapping match the value of the `expression`, then the content
|
|
* of the `other` key is returned when present, otherwise an empty string is returned.
|
|
*
|
|
* ## Example
|
|
*
|
|
* {@example common/pipes/ts/i18n_pipe.ts region='I18nSelectPipeComponent'}
|
|
*
|
|
* @experimental
|
|
*/
|
|
@Pipe({name: 'i18nSelect', pure: true})
|
|
export class I18nSelectPipe implements PipeTransform {
|
|
transform(value: string, mapping: {[key: string]: string}): string {
|
|
if (value == null) return '';
|
|
|
|
if (typeof mapping !== 'object' || typeof value !== 'string') {
|
|
throw invalidPipeArgumentError(I18nSelectPipe, mapping);
|
|
}
|
|
|
|
if (mapping.hasOwnProperty(value)) {
|
|
return mapping[value];
|
|
}
|
|
|
|
if (mapping.hasOwnProperty('other')) {
|
|
return mapping['other'];
|
|
}
|
|
|
|
return '';
|
|
}
|
|
}
|