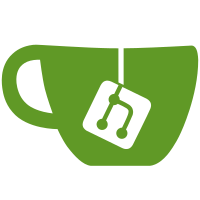
Major changes: - `compiler.compileRoot(el, type)` -> `compiler.compileInHost(type) + viewHydrator.hydrateHostViewInPlace(el, view)` - move all `hydrate`/`dehydrate` methods out of `View` and `ViewContainer` into a standalone class `view_hydrator` as private methods and provide new public methods dedicated to the individual use cases. Note: This PR does not change the current functionality, only moves it into different places. See design discussion in #1351, in preparation for imperative views.
36 lines
1.1 KiB
JavaScript
36 lines
1.1 KiB
JavaScript
import {isPresent} from 'angular2/src/facade/lang';
|
|
import {DOM} from 'angular2/src/dom/dom_adapter';
|
|
|
|
import {List, Map, ListWrapper, MapWrapper} from 'angular2/src/facade/collection';
|
|
|
|
import {ElementBinder} from './element_binder';
|
|
import {NG_BINDING_CLASS} from '../util';
|
|
|
|
export class RenderProtoView {
|
|
element;
|
|
elementBinders:List<ElementBinder>;
|
|
isTemplateElement:boolean;
|
|
rootBindingOffset:int;
|
|
|
|
constructor({
|
|
elementBinders,
|
|
element
|
|
}) {
|
|
this.element = element;
|
|
this.elementBinders = elementBinders;
|
|
this.isTemplateElement = DOM.isTemplateElement(this.element);
|
|
this.rootBindingOffset = (isPresent(this.element) && DOM.hasClass(this.element, NG_BINDING_CLASS)) ? 1 : 0;
|
|
}
|
|
|
|
mergeChildComponentProtoViews(componentProtoViews:List<RenderProtoView>) {
|
|
var componentProtoViewIndex = 0;
|
|
for (var i=0; i<this.elementBinders.length; i++) {
|
|
var eb = this.elementBinders[i];
|
|
if (isPresent(eb.componentId)) {
|
|
eb.nestedProtoView = componentProtoViews[componentProtoViewIndex];
|
|
componentProtoViewIndex++;
|
|
}
|
|
}
|
|
}
|
|
}
|