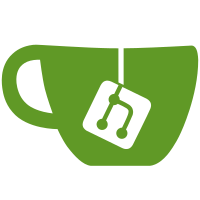
Add a transformer for `di` which generates `.ng_deps.dart` files for all `.dart` files it is run on. These `.ng_deps.dart` files register metadata for any `@Injectable` classes. Fix unit tests for changes introduced by the di transformer. When using `pub (build|serve) --mode=ngstatic`, we will also generate getters and setters, parse templates, and remove import of `dart:mirrors` in the Angular transform. Because this is still relatively immature, we use the mode to keep it opt-in for now. Closes #700
46 lines
1.5 KiB
Dart
46 lines
1.5 KiB
Dart
library angular2.transform;
|
|
|
|
import 'package:barback/barback.dart';
|
|
import 'package:dart_style/dart_style.dart';
|
|
|
|
import 'directive_linker/transformer.dart';
|
|
import 'directive_processor/transformer.dart';
|
|
import 'bind_generator/transformer.dart';
|
|
import 'reflection_remover/transformer.dart';
|
|
import 'template_compiler/transformer.dart';
|
|
import 'common/formatter.dart' as formatter;
|
|
import 'common/names.dart';
|
|
import 'common/options.dart';
|
|
|
|
export 'common/options.dart';
|
|
|
|
/// Replaces Angular 2 mirror use with generated code.
|
|
class AngularTransformerGroup extends TransformerGroup {
|
|
AngularTransformerGroup._(phases) : super(phases) {
|
|
formatter.init(new DartFormatter());
|
|
}
|
|
|
|
factory AngularTransformerGroup(TransformerOptions options) {
|
|
var phases = [[new DirectiveProcessor(options)], [new DirectiveLinker()]];
|
|
if (options.modeName == TRANSFORM_MODE) {
|
|
phases.addAll([
|
|
[new BindGenerator(options)],
|
|
[new TemplateComplier(options)],
|
|
[new ReflectionRemover(options)]
|
|
]);
|
|
}
|
|
return new AngularTransformerGroup._(phases);
|
|
}
|
|
|
|
factory AngularTransformerGroup.asPlugin(BarbackSettings settings) {
|
|
return new AngularTransformerGroup(_parseOptions(settings));
|
|
}
|
|
}
|
|
|
|
TransformerOptions _parseOptions(BarbackSettings settings) {
|
|
var config = settings.configuration;
|
|
return new TransformerOptions(config[ENTRY_POINT_PARAM],
|
|
reflectionEntryPoint: config[REFLECTION_ENTRY_POINT_PARAM],
|
|
modeName: settings.mode.name);
|
|
}
|