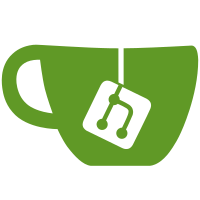
When testing JIT code, it is useful to be able to access the generated JIT source. Previously this is done by spying on the global `Function` object, to capture the code when it is being evaluated. This is problematic because you can only capture the body of the function, and not the arguments, which messes up line and column positions for source mapping for instance. Now the code that generates and then evaluates JIT code is wrapped in a `JitEvaluator` class, making it possible to provide a mock implementation that can capture the generated source of the function passed to `executeFunction(fn: Function, args: any[])`. PR Close #28055
49 lines
1.8 KiB
TypeScript
49 lines
1.8 KiB
TypeScript
/**
|
|
* @license
|
|
* Copyright Google Inc. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
import {CompileReflector} from '../compile_reflector';
|
|
import * as o from '../output/output_ast';
|
|
|
|
/**
|
|
* Implementation of `CompileReflector` which resolves references to @angular/core
|
|
* symbols at runtime, according to a consumer-provided mapping.
|
|
*
|
|
* Only supports `resolveExternalReference`, all other methods throw.
|
|
*/
|
|
export class R3JitReflector implements CompileReflector {
|
|
constructor(private context: {[key: string]: any}) {}
|
|
|
|
resolveExternalReference(ref: o.ExternalReference): any {
|
|
// This reflector only handles @angular/core imports.
|
|
if (ref.moduleName !== '@angular/core') {
|
|
throw new Error(
|
|
`Cannot resolve external reference to ${ref.moduleName}, only references to @angular/core are supported.`);
|
|
}
|
|
if (!this.context.hasOwnProperty(ref.name !)) {
|
|
throw new Error(`No value provided for @angular/core symbol '${ref.name!}'.`);
|
|
}
|
|
return this.context[ref.name !];
|
|
}
|
|
|
|
parameters(typeOrFunc: any): any[][] { throw new Error('Not implemented.'); }
|
|
|
|
annotations(typeOrFunc: any): any[] { throw new Error('Not implemented.'); }
|
|
|
|
shallowAnnotations(typeOrFunc: any): any[] { throw new Error('Not implemented.'); }
|
|
|
|
tryAnnotations(typeOrFunc: any): any[] { throw new Error('Not implemented.'); }
|
|
|
|
propMetadata(typeOrFunc: any): {[key: string]: any[];} { throw new Error('Not implemented.'); }
|
|
|
|
hasLifecycleHook(type: any, lcProperty: string): boolean { throw new Error('Not implemented.'); }
|
|
|
|
guards(typeOrFunc: any): {[key: string]: any;} { throw new Error('Not implemented.'); }
|
|
|
|
componentModuleUrl(type: any, cmpMetadata: any): string { throw new Error('Not implemented.'); }
|
|
}
|