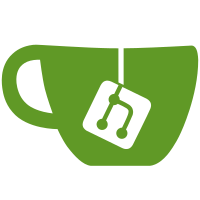
BREAKING CHANGES: Dart applications and TypeScript applications meant to transpile to Dart must now import `package:angular2/bootstrap.dart` instead of `package:angular2/angular2.dart` in their bootstrap code. `package:angular2/angular2.dart` no longer export the bootstrap function. The transformer rewrites imports of `bootstrap.dart` and calls to `bootstrap` to `bootstrap_static.dart` and `bootstrapStatic` respectively.
44 lines
1.4 KiB
Dart
44 lines
1.4 KiB
Dart
library angular2.transform.reflection_remover.ast_tester;
|
|
|
|
import 'package:analyzer/src/generated/ast.dart';
|
|
import 'package:analyzer/src/generated/element.dart';
|
|
import 'package:angular2/src/transform/common/names.dart';
|
|
|
|
/// An object that checks for {@link ReflectionCapabilities} syntactically, that is,
|
|
/// without resolution information.
|
|
class AstTester {
|
|
const AstTester();
|
|
|
|
bool isNewReflectionCapabilities(InstanceCreationExpression node) =>
|
|
'${node.constructorName.type.name}' == REFLECTION_CAPABILITIES_NAME;
|
|
|
|
bool isReflectionCapabilitiesImport(ImportDirective node) {
|
|
return node.uri.stringValue.endsWith("reflection_capabilities.dart");
|
|
}
|
|
|
|
bool isBootstrapImport(ImportDirective node) {
|
|
return node.uri.stringValue.endsWith("/bootstrap.dart");
|
|
}
|
|
}
|
|
|
|
/// An object that checks for {@link ReflectionCapabilities} using a fully resolved
|
|
/// Ast.
|
|
class ResolvedTester implements AstTester {
|
|
final ClassElement _forbiddenClass;
|
|
|
|
ResolvedTester(this._forbiddenClass);
|
|
|
|
bool isNewReflectionCapabilities(InstanceCreationExpression node) {
|
|
var typeElement = node.constructorName.type.name.bestElement;
|
|
return typeElement != null && typeElement == _forbiddenClass;
|
|
}
|
|
|
|
bool isReflectionCapabilitiesImport(ImportDirective node) {
|
|
return node.uriElement == _forbiddenClass.library;
|
|
}
|
|
|
|
bool isBootstrapImport(ImportDirective node) {
|
|
throw 'Not implemented';
|
|
}
|
|
}
|