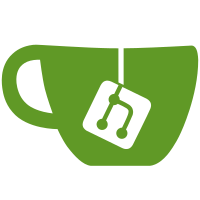
With #31953 we moved the factories for components, directives and pipes into a new field called `ngFactoryDef`, however I decided not to do it for injectables, because they needed some extra logic. These changes set up the `ngFactoryDef` for injectables as well. For reference, the extra logic mentioned above is that for injectables we have two code paths: 1. For injectables that don't configure how they should be instantiated, we create a `factory` that proxies to `ngFactoryDef`: ``` // Source @Injectable() class Service {} // Output class Service { static ngInjectableDef = defineInjectable({ factory: () => Service.ngFactoryFn(), }); static ngFactoryFn: (t) => new (t || Service)(); } ``` 2. For injectables that do configure how they're created, we keep the `ngFactoryDef` and generate the factory based on the metadata: ``` // Source @Injectable({ useValue: DEFAULT_IMPL, }) class Service {} // Output export class Service { static ngInjectableDef = defineInjectable({ factory: () => DEFAULT_IMPL, }); static ngFactoryFn: (t) => new (t || Service)(); } ``` PR Close #32433
33 lines
880 B
TypeScript
33 lines
880 B
TypeScript
/**
|
|
* @license
|
|
* Copyright Google Inc. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
import {Pipe, PipeTransform} from '@angular/core';
|
|
|
|
/**
|
|
* @ngModule CommonModule
|
|
* @description
|
|
*
|
|
* Converts a value into its JSON-format representation. Useful for debugging.
|
|
*
|
|
* @usageNotes
|
|
*
|
|
* The following component uses a JSON pipe to convert an object
|
|
* to JSON format, and displays the string in both formats for comparison.
|
|
*
|
|
* {@example common/pipes/ts/json_pipe.ts region='JsonPipe'}
|
|
*
|
|
* @publicApi
|
|
*/
|
|
@Pipe({name: 'json', pure: false})
|
|
export class JsonPipe implements PipeTransform {
|
|
/**
|
|
* @param value A value of any type to convert into a JSON-format string.
|
|
*/
|
|
transform(value: any): string { return JSON.stringify(value, null, 2); }
|
|
}
|