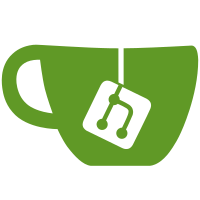
DEPRECATION: - the arguments `inputs` / `outputs` / `ngContentSelectors` of `downgradeComponent` are no longer used as Angular calculates these automatically now. - Compiler.getNgContentSelectors is deprecated. Use ComponentFactory.ngContentSelectors instead.
35 lines
1.0 KiB
TypeScript
35 lines
1.0 KiB
TypeScript
/**
|
|
* @license
|
|
* Copyright Google Inc. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
/**
|
|
* A `PropertyBinding` represents a mapping between a property name
|
|
* and an attribute name. It is parsed from a string of the form
|
|
* `"prop: attr"`; or simply `"propAndAttr" where the property
|
|
* and attribute have the same identifier.
|
|
*/
|
|
export class PropertyBinding {
|
|
bracketAttr: string;
|
|
bracketParenAttr: string;
|
|
parenAttr: string;
|
|
onAttr: string;
|
|
bindAttr: string;
|
|
bindonAttr: string;
|
|
|
|
constructor(public prop: string, public attr: string) { this.parseBinding(); }
|
|
|
|
private parseBinding() {
|
|
this.bracketAttr = `[${this.attr}]`;
|
|
this.parenAttr = `(${this.attr})`;
|
|
this.bracketParenAttr = `[(${this.attr})]`;
|
|
const capitalAttr = this.attr.charAt(0).toUpperCase() + this.attr.substr(1);
|
|
this.onAttr = `on${capitalAttr}`;
|
|
this.bindAttr = `bind${capitalAttr}`;
|
|
this.bindonAttr = `bindon${capitalAttr}`;
|
|
}
|
|
}
|