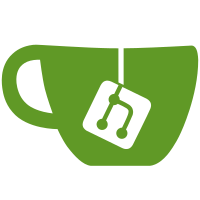
This commit adds the `AstHost` interface, along with implementations for both Babel and TS. It also implements the Babel vesion of the `AstFactory` interface, along with a linker specific implementation of the `ImportGenerator` interface. These classes will be used by the new "ng-linker" to transform prelinked library code using a Babel plugin. PR Close #38866
38 lines
1.3 KiB
TypeScript
38 lines
1.3 KiB
TypeScript
/**
|
|
* @license
|
|
* Copyright Google LLC All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
import {ImportGenerator, NamedImport} from '../../src/ngtsc/translator';
|
|
|
|
/**
|
|
* A class that is used to generate imports when translating from Angular Output AST to an AST to
|
|
* render, such as Babel.
|
|
*
|
|
* Note that, in the linker, there can only be imports from `@angular/core` and that these imports
|
|
* must be achieved by property access on an `ng` namespace identifer, which is passed in via the
|
|
* constructor.
|
|
*/
|
|
export class LinkerImportGenerator<TExpression> implements ImportGenerator<TExpression> {
|
|
constructor(private ngImport: TExpression) {}
|
|
|
|
generateNamespaceImport(moduleName: string): TExpression {
|
|
this.assertModuleName(moduleName);
|
|
return this.ngImport;
|
|
}
|
|
|
|
generateNamedImport(moduleName: string, originalSymbol: string): NamedImport<TExpression> {
|
|
this.assertModuleName(moduleName);
|
|
return {moduleImport: this.ngImport, symbol: originalSymbol};
|
|
}
|
|
|
|
private assertModuleName(moduleName: string): void {
|
|
if (moduleName !== '@angular/core') {
|
|
throw new Error(`Unable to import from anything other than '@angular/core'`);
|
|
}
|
|
}
|
|
}
|