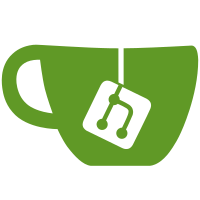
Provides a runtime and compile time switch for ivy including `ApplicationRef.bootstrapModule`. This is done by naming the symbols such that `ngcc` (angular Compatibility compiler) can rename symbols in such a way that running `ngcc` command will switch the `@angular/core` module from `legacy` to `ivy` mode. This is done as follows: ``` const someToken__PRE_NGCC__ = ‘legacy mode’; const someToken__POST_NGCC__ = ‘ivy mode’; export someSymbol = someToken__PRE_NGCC__; ``` The `ngcc` will search for any token which ends with `__PRE_NGCC__` and replace it with `__POST_NGCC__`. This allows the `@angular/core` package to be rewritten to ivy mode post `ngcc` execution. PR Close #25238
46 lines
1.3 KiB
TypeScript
46 lines
1.3 KiB
TypeScript
/**
|
|
* @license
|
|
* Copyright Google Inc. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
/**
|
|
* This file is used to control if the default rendering pipeline should be `ViewEngine` or `Ivy`.
|
|
*
|
|
* For more information on how to run and debug tests with either Ivy or View Engine (legacy),
|
|
* please see [BAZEL.md](./docs/BAZEL.md).
|
|
*/
|
|
|
|
let _devMode: boolean = true;
|
|
let _runModeLocked: boolean = false;
|
|
|
|
|
|
/**
|
|
* Returns whether Angular is in development mode. After called once,
|
|
* the value is locked and won't change any more.
|
|
*
|
|
* By default, this is true, unless a user calls `enableProdMode` before calling this.
|
|
*
|
|
* @experimental APIs related to application bootstrap are currently under review.
|
|
*/
|
|
export function isDevMode(): boolean {
|
|
_runModeLocked = true;
|
|
return _devMode;
|
|
}
|
|
|
|
/**
|
|
* Disable Angular's development mode, which turns off assertions and other
|
|
* checks within the framework.
|
|
*
|
|
* One important assertion this disables verifies that a change detection pass
|
|
* does not result in additional changes to any bindings (also known as
|
|
* unidirectional data flow).
|
|
*/
|
|
export function enableProdMode(): void {
|
|
if (_runModeLocked) {
|
|
throw new Error('Cannot enable prod mode after platform setup.');
|
|
}
|
|
_devMode = false;
|
|
} |