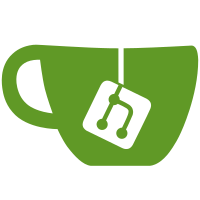
BREAKING CHANGE: Replace @Ancestor() with @Host() @SkipSelf() Replace @Unbounded() wwith @SkipSelf() Replace @Ancestor({self:true}) with @Host() Replace @Unbounded({self:true}) with nothing Replace new AncestorMetadata() with [new HostMetadata(), new SkipSelfMetadata()] Replace new UnboundedMetadata() with new SkipSelfMetadata() Replace new Ancestor({self:true}) with new HostMetadata()
87 lines
1.8 KiB
TypeScript
87 lines
1.8 KiB
TypeScript
import {
|
|
InjectMetadata,
|
|
OptionalMetadata,
|
|
InjectableMetadata,
|
|
SelfMetadata,
|
|
HostMetadata,
|
|
SkipSelfMetadata
|
|
} from './metadata';
|
|
import {makeDecorator, makeParamDecorator, TypeDecorator} from '../util/decorators';
|
|
|
|
/**
|
|
* Factory for creating {@link InjectMetadata}.
|
|
*/
|
|
export interface InjectFactory {
|
|
(token: any): any;
|
|
new (token: any): InjectMetadata;
|
|
}
|
|
|
|
/**
|
|
* Factory for creating {@link OptionalMetadata}.
|
|
*/
|
|
export interface OptionalFactory {
|
|
(): any;
|
|
new (): OptionalMetadata;
|
|
}
|
|
|
|
/**
|
|
* Factory for creating {@link InjectableMetadata}.
|
|
*/
|
|
export interface InjectableFactory {
|
|
(): any;
|
|
new (): InjectableMetadata;
|
|
}
|
|
|
|
/**
|
|
* Factory for creating {@link SelfMetadata}.
|
|
*/
|
|
export interface SelfFactory {
|
|
(): any;
|
|
new (): SelfMetadata;
|
|
}
|
|
|
|
/**
|
|
* Factory for creating {@link HostMetadata}.
|
|
*/
|
|
export interface HostFactory {
|
|
(): any;
|
|
new (): HostMetadata;
|
|
}
|
|
|
|
/**
|
|
* Factory for creating {@link SkipSelfMetadata}.
|
|
*/
|
|
export interface SkipSelfFactory {
|
|
(): any;
|
|
new (): SkipSelfMetadata;
|
|
}
|
|
|
|
/**
|
|
* Factory for creating {@link InjectMetadata}.
|
|
*/
|
|
export var Inject: InjectFactory = makeParamDecorator(InjectMetadata);
|
|
|
|
/**
|
|
* Factory for creating {@link OptionalMetadata}.
|
|
*/
|
|
export var Optional: OptionalFactory = makeParamDecorator(OptionalMetadata);
|
|
|
|
/**
|
|
* Factory for creating {@link InjectableMetadata}.
|
|
*/
|
|
export var Injectable: InjectableFactory = <InjectableFactory>makeDecorator(InjectableMetadata);
|
|
|
|
/**
|
|
* Factory for creating {@link SelfMetadata}.
|
|
*/
|
|
export var Self: SelfFactory = makeParamDecorator(SelfMetadata);
|
|
|
|
/**
|
|
* Factory for creating {@link HostMetadata}.
|
|
*/
|
|
export var Host: HostFactory = makeParamDecorator(HostMetadata);
|
|
|
|
/**
|
|
* Factory for creating {@link SkipSelfMetadata}.
|
|
*/
|
|
export var SkipSelf: SkipSelfFactory = makeParamDecorator(SkipSelfMetadata); |