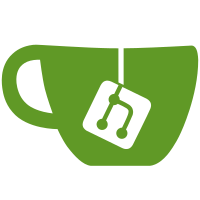
Adds a command for building all release packages. This command is primarily used by the release tool for building release output in version branches. The release tool cannot build the release packages configured in `master` as those packages could differ from the packages available in a given version branch. Also, the build process could have changed, so we want to have an API for building release packages that is guaranteed to be consistent across branches. PR Close #38656
27 lines
764 B
TypeScript
27 lines
764 B
TypeScript
/**
|
|
* @license
|
|
* Copyright Google LLC All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
import * as yargs from 'yargs';
|
|
|
|
import {ReleaseBuildCommandModule} from './build/cli';
|
|
import {buildEnvStamp} from './stamping/env-stamp';
|
|
|
|
/** Build the parser for the release commands. */
|
|
export function buildReleaseParser(localYargs: yargs.Argv) {
|
|
return localYargs.help()
|
|
.strict()
|
|
.demandCommand()
|
|
.command(ReleaseBuildCommandModule)
|
|
.command(
|
|
'build-env-stamp', 'Build the environment stamping information', {},
|
|
() => buildEnvStamp());
|
|
}
|
|
|
|
if (require.main === module) {
|
|
buildReleaseParser(yargs).parse();
|
|
}
|