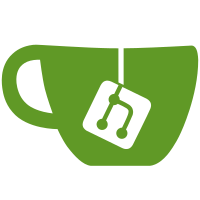
This affects the dynamic version of `upgrade` and makes it more consistent with the static version, while removing an artificial limitation. This commit also refactors the file layout and code, in order to share code wrt to dowgrading components between the dynamic and static versions.
53 lines
2.2 KiB
TypeScript
53 lines
2.2 KiB
TypeScript
/**
|
|
* @license
|
|
* Copyright Google Inc. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
import {PropertyBinding} from '@angular/upgrade/src/common/component_info';
|
|
|
|
export function main() {
|
|
describe('PropertyBinding', () => {
|
|
it('should process a simple binding', () => {
|
|
const binding = new PropertyBinding('someBinding');
|
|
expect(binding.binding).toEqual('someBinding');
|
|
expect(binding.prop).toEqual('someBinding');
|
|
expect(binding.attr).toEqual('someBinding');
|
|
expect(binding.bracketAttr).toEqual('[someBinding]');
|
|
expect(binding.bracketParenAttr).toEqual('[(someBinding)]');
|
|
expect(binding.parenAttr).toEqual('(someBinding)');
|
|
expect(binding.onAttr).toEqual('onSomeBinding');
|
|
expect(binding.bindAttr).toEqual('bindSomeBinding');
|
|
expect(binding.bindonAttr).toEqual('bindonSomeBinding');
|
|
});
|
|
|
|
it('should process a two-part binding', () => {
|
|
const binding = new PropertyBinding('someProp:someAttr');
|
|
expect(binding.binding).toEqual('someProp:someAttr');
|
|
expect(binding.prop).toEqual('someProp');
|
|
expect(binding.attr).toEqual('someAttr');
|
|
expect(binding.bracketAttr).toEqual('[someAttr]');
|
|
expect(binding.bracketParenAttr).toEqual('[(someAttr)]');
|
|
expect(binding.parenAttr).toEqual('(someAttr)');
|
|
expect(binding.onAttr).toEqual('onSomeAttr');
|
|
expect(binding.bindAttr).toEqual('bindSomeAttr');
|
|
expect(binding.bindonAttr).toEqual('bindonSomeAttr');
|
|
});
|
|
|
|
it('should cope with whitespace', () => {
|
|
const binding = new PropertyBinding(' someProp : someAttr ');
|
|
expect(binding.binding).toEqual(' someProp : someAttr ');
|
|
expect(binding.prop).toEqual('someProp');
|
|
expect(binding.attr).toEqual('someAttr');
|
|
expect(binding.bracketAttr).toEqual('[someAttr]');
|
|
expect(binding.bracketParenAttr).toEqual('[(someAttr)]');
|
|
expect(binding.parenAttr).toEqual('(someAttr)');
|
|
expect(binding.onAttr).toEqual('onSomeAttr');
|
|
expect(binding.bindAttr).toEqual('bindSomeAttr');
|
|
expect(binding.bindonAttr).toEqual('bindonSomeAttr');
|
|
});
|
|
});
|
|
}
|