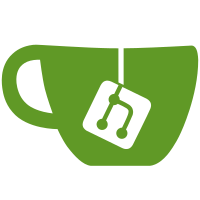
- updates tests - heavy prose revisions - uses HttpClient (with angular-in-memory-web-api) - test HeroService using `HttpClientTestingModule` - scrub away most By.CSS - fake async observable with `asyncData()` - extensive Twain work - different take on retryWhen - remove app barrels (& systemjs.extras) which troubled plunker/systemjs - add dummy export const to hero.ts (plunkr/systemjs fails w/o it) - shrink and re-organize TOC - add marble testing package and tests - demonstrate the "no beforeEach()" test coding style - add section on Http service testing - prepare for stackblitz - confirm works in plunker except excluded marble test - add tests for avoidFile class feature of CodeExampleComponent PR Close #20697
51 lines
1.6 KiB
TypeScript
51 lines
1.6 KiB
TypeScript
import { NgModule } from '@angular/core';
|
|
import { BrowserModule } from '@angular/platform-browser';
|
|
import { HttpClientModule } from '@angular/common/http';
|
|
|
|
import { AppComponent } from './app.component';
|
|
import { AppRoutingModule } from './app-routing.module';
|
|
|
|
import { AboutComponent } from './about/about.component';
|
|
import { BannerComponent } from './banner/banner.component';
|
|
import { HeroService } from './model/hero.service';
|
|
import { UserService } from './model/user.service';
|
|
import { TwainComponent } from './twain/twain.component';
|
|
import { TwainService } from './twain/twain.service';
|
|
import { WelcomeComponent } from './welcome/welcome.component';
|
|
|
|
import { DashboardModule } from './dashboard/dashboard.module';
|
|
import { SharedModule } from './shared/shared.module';
|
|
|
|
import { HttpClientInMemoryWebApiModule } from 'angular-in-memory-web-api';
|
|
import { InMemoryDataService } from './in-memory-data.service';
|
|
|
|
@NgModule({
|
|
imports: [
|
|
BrowserModule,
|
|
DashboardModule,
|
|
AppRoutingModule,
|
|
SharedModule,
|
|
HttpClientModule,
|
|
|
|
// The HttpClientInMemoryWebApiModule module intercepts HTTP requests
|
|
// and returns simulated server responses.
|
|
// Remove it when a real server is ready to receive requests.
|
|
HttpClientInMemoryWebApiModule.forRoot(
|
|
InMemoryDataService, { dataEncapsulation: false }
|
|
)
|
|
],
|
|
providers: [
|
|
HeroService,
|
|
TwainService,
|
|
UserService
|
|
],
|
|
declarations: [
|
|
AppComponent,
|
|
AboutComponent,
|
|
BannerComponent,
|
|
TwainComponent,
|
|
WelcomeComponent ],
|
|
bootstrap: [ AppComponent ]
|
|
})
|
|
export class AppModule { }
|