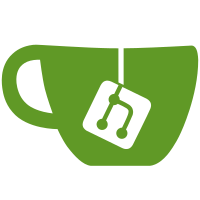
Structural directives can now specify a type guard that describes what types can be inferred for an input expression inside the directive's template. NgIf was modified to declare an input guard on ngIf. After this change, `fullTemplateTypeCheck` will infer that usage of `ngIf` expression inside it's template is truthy. For example, if a component has a property `person?: Person` and a template of `<div *ngIf="person"> {{person.name}} </div>` the compiler will no longer report that `person` might be null or undefined. The template compiler will generate code similar to, ``` if (NgIf.ngIfTypeGuard(instance.person)) { instance.person.name } ``` to validate the template's use of the interpolation expression. Calling the type guard in this fashion allows TypeScript to infer that `person` is non-null. Fixes: #19756? PR Close #20702
24 lines
888 B
TypeScript
24 lines
888 B
TypeScript
/**
|
|
* @license
|
|
* Copyright Google Inc. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by an MIT-style license that can be
|
|
* found in the LICENSE file at https://angular.io/license
|
|
*/
|
|
|
|
import {Component} from './core';
|
|
import * as o from './output/output_ast';
|
|
|
|
/**
|
|
* Provides access to reflection data about symbols that the compiler needs.
|
|
*/
|
|
export abstract class CompileReflector {
|
|
abstract parameters(typeOrFunc: /*Type*/ any): any[][];
|
|
abstract annotations(typeOrFunc: /*Type*/ any): any[];
|
|
abstract propMetadata(typeOrFunc: /*Type*/ any): {[key: string]: any[]};
|
|
abstract hasLifecycleHook(type: any, lcProperty: string): boolean;
|
|
abstract guards(typeOrFunc: /* Type */ any): {[key: string]: any};
|
|
abstract componentModuleUrl(type: /*Type*/ any, cmpMetadata: Component): string;
|
|
abstract resolveExternalReference(ref: o.ExternalReference): any;
|
|
}
|